Whether you’re still learning Javascript or are an advanced developer, it can be helpful to keep a reference sheet for common JavaScript commands. We’ve compiled a downloadable JavaScript cheat sheet to keep near your desk.
But first, let’s recap what JavaScript is and how it works.
What is JavaScript?
JavaScript is a programming language used to create interactive elements of a website, like maps, scrolling videos, and animation. It works in tandem with other coding languages, like HTML and CSS.
What is the Difference Between JavaScript, HTML, and CSS?
JavaScript, HTML, and CSS are all programming languages with major differences:
HTML is the language used to define text and headings, as well as embed images on a webpage. CSS is the language used to apply styling to the HTML content, like colors and fonts. JavaScript is the language that creates interactive and dynamic content like moving graphics, maps, scrolling videos, and more.Where JavaScript Sits on Your Website
JavaScript sits in the HTML of your webpages. It needs to be bookended by <script> tags. For example:
<script type="text/javascript">//JS code goes here</script>You can also add your JavaScript to a file and link to it within your HTML code. This form of linking, referred to sometimes as “linking JavaScript externally,” helps you keep track of your code.
How to Write JavaScript
There are categories of JavaScript code, including:
Variables: A variable is an element that can vary (i.e. a data value that can be changed.) Operators: As with other programming languages, an operator operates single or multiple data values to produce a result. Functions: A function takes an input and produces a related output. This is usually a set of statements that performs a task to produce a result. Loops: This loops a block of code until certain conditions are met. If - Else Statements: Like a flowchart, If-Else Statements run blocks of code if certain conditions are true. If the conditions are false, another block of code is run. Strings: Strings are a way to store text. In strings, the first character is 0, the second character is 1, the third character is 2, and so on. Regular Expressions: Also called a rational expression, a regular expression is a string of characters in a pattern used to match character combinations. Numbers and Math: Numbers and Math are static properties for mathematical constants and functions. Dates: As the name suggests, dates in JavaScript are a moment in time that can be as specific as a particular millisecond on any particular day. DOM Node: A DOM node is a way to allow JavaScript to dynamically update the content of a page. Events: An event is any change or update to the webpage that occurs as of JavaScript code being run.JavaScript Command Cheat Sheet
When working with JavaScript, try our complete ‘cheat sheet’ of JavaScript commands.
Variables
These are elements that can vary, which can include variables, data types, and arrays.
var
The most common variable. Var variables move to the top when code is executed
const
A variable that cannot be reassigned and is not accessible before they appear within the code
let
The let variable can be reassigned but not re-declared
var age = 23
Numbers
var x
Variables
var a = "init"
Text (strings)
var b = 1 + 2 + 3
Operations
var c = true
True or false statements
const PI = 3.14
Constant numbers
var name = {firstName:"John", lastName:”Doe"}
Objects
concat()
Join several arrays into one
indexOf()
Returns the first position at which a given element appears in an array
join()
Combine elements of an array into a single string and return the string
lastIndexOf()
Gives the last position at which a given element appears in an array
pop()
Removes the last element of an array
push()
Add a new element at the end
reverse()
Reverse the order of the elements in an array
shift()
Remove the first element of an array
slice()
Pulls a copy of a portion of an array into a new array of 4 24
sort()
Sorts elements alphabetically
splice()
Adds elements in a specified way and position
toString()
Converts elements to strings
unshift()
Adds a new element to the beginning
valueOf()
Returns the primitive value of the specified object
Operators
These are single or multiple data values used to produce a result, including Basic Operators, Comparison Operators, Logical Operators, and Bitwise Operators.
(plus sign) +
Addition
-
Subtraction
*
Multiplication
/
Division
(..) Grouping operator
Grouping operator
%
Remainder
(two plus signs) ++
Increment numbers
--
Decrement numbers
( 2 equal signs) ==
Equal to
(3 equal signs) ===
Equal value and equal type
!=
Not equal
!==
Not equal value or not equal type
>
Greater than
<
Less than
>=
Greater than or equal to
<=
Less than or equal to
?
Ternary operator
&&
Logical and
!!
Logical or
!
Logical not
&
AND statement
|
OR statement
~
NOT
^
XOR
<<
Left shift
>>
Right shift
>>>
Zero fill right shift
Functions
These perform a task to produce a related output, including Outputting Data and Global Functions.
alert()
An alert box is displayed with an OK button
confirm()
Displays a message box with ok/cancel options
console.log()
Writes information to the browser
document.write()
Write directly to the HTML document
prompt()
A pop up box that needs user input
decodeURI()
Decodes a Uniform Resource Identifier (URI) created by encodeURI
decodeURIComponent()
Decodes a URI component
encodeURI()
Encodes a URI into UTF-8
encodeURIComponent()
Encodes a URI using numbers to represent letters
eval()
Evaluates JavaScript code represented as a string
isFinite()
Determines whether a passed value is a finite number
isNaN()
Determines whether a value is NaN or not
Number()
Returns a number converted from its argument
parseFloat()
Parses an argument and returns a floating point number
parseInt()
Parses its argument and returns an integer
Loops
These occur until certain conditions are met.
for
The most common way to create a loop in JavaScript
while
Sets up conditions for a loop
do-while
Checks once again to see if the conditions are met
break
Stops the loop cycle if conditions aren’t met
continue
Skip parts of the loop if conditions are met
If - Else Statements
These are designed to run blocks of code if certain conditions are met.
if (condition) {//}
If a condition is met do this
} else {//}
If a condition is not met do this
Strings
These store text and include escape characters and string methods.
\'
Single quote
\"
Double quote
\\
Backslash
\b
Backspace
\f
Form feed
\n
Newline
\r
Carriage return
\t
Horizontal tabulator
\v
Vertical tabulator
charAt()
Returns a character at a specified position
charCodeAt()
Gives you the unicode of character at a specified position
concat()
Joins multiple strings together
fromCharCode()
Converts UTF-16 unicode values to characters
indexOf()
Returns the position of the first occurrence of a specified element
lastIndexOf()
Returns the position of the last occurrence of a specified element
match()
Returns any matches of a string
replace()
Find and replace specific text
search()
Searches for text and returns its position
slice()
Extracts a section of a string and returns it
split()
Splits a string into substrings and returns it at a specified position
substr()
Extracts a string and returns it at a specified position
substring()
Excluding negative indices, splits a string into substrings and returns it at a specified position
toLowerCase()
Convert to lowercase
toUpperCase()
Convert to uppercase
valueOf()
Returns the primitive value of a string object
Regular Expressions
These are patterns, including pattern modifiers, brackets, metacharacters, and quantifiers.
e
Evaluate replacement
i
Perform case-insensitive matching
g
Perform global matching
m
Perform multiple line matching
s
Treat strings as a single line
x
Allow comments and whitespace in pattern
U
Non Greedy pattern
[abc]
Find any of the characters between the brackets
[^abc]
Find any character not in the brackets
[0-9]
Used to find any digit from 0 to 9
[A-z]
Find any character from uppercase A to lowercase z
(a|b|c)
Find any of the alternatives separated with |
.
Find a single character, except newline or line terminator
\w
Word character
\W
Non-word character
\d
A digit
\D
A non-digit character
\s
Whitespace character
\S
Non-whitespace character
\b
Find a match at the beginning/end of a word
\B
A match not at the beginning/end of a word
\0
NUL character
\n
A new line character
\f
Form feed character
\r
Carriage return character
\t
Tab character
\v
Vertical tab character
\xxx
The character specified by an octal number xxx
\xdd
The character specified by a hexadecimal number dd
\uxxxx
The Unicode character specified by a hexadecimal number xxxx
n+
Matches any string that contains at least one n
Numbers and Maths
These are mathematical functions, including number properties, number methods, math properties, and math methods.
MAX_VALUE
The maximum numeric value representable in JavaScript
MIN_VALUE
Smallest positive numeric value representable in JavaScript
NaN
The “Not-a-Number” value
NEGATIVE_INFINITY
The negative Infinity value
POSITIVE_INFINITY
Positive Infinity value
toExponential()
Returns a string with a rounded number written as exponential notation
toFixed()
Returns the string of a number with a specified number of decimals
toPrecision()
A string of a number written with a specified length
toString()
Returns a number as a string
valueOf()
Returns a number as a number
E
Euler’s number
LN2
The natural logarithm of 2
LN10
Natural logarithm of 10
LOG2E
Base 2 logarithm of E
LOG10E
Base 10 logarithm of E
PI
The number PI
SQRT1_2
The square root of 1/2
SQRT2
The square root of 2
abs(x)
Returns the absolute (positive) value of x
Dates
These include specific points in time and include setting dates, and pulling date and time values, and set part of a date.
tan(x)
The tangent of an angle
Date()
Creates a new date object with the current date and time
Date(2017, 5, 21, 3, 23, 10, 0)
Create a custom date object. The numbers represent year, month, day, hour, minutes, seconds, milliseconds. You can omit anything you want except for year and month.
Date("2017-06-23")
Date declaration as a string
getDate()
Get the day of the month as a number (1-31)
getDay()
The weekday as a number (0-6)
getFullYear()
Year as a four digit number (yyyy)
getHours()
Get the hour (0-23)
getMilliseconds()
The millisecond (0-999)
getMinutes()
Get the minute (0-59)
getMonth()
Month as a number (0-11)
getSeconds()
Get the second (0-59)
getTime()
Get the milliseconds since January 1, 1970
getUTCDate()
The day (date) of the month in the specified date according to universal time (also available for day, month, full year, hours, minutes etc.)
parse
Parses a string representation of a date, and returns the number of milliseconds since January 1, 1970
setDate()
Set the day as a number (1-31)
setFullYear()
Sets the year (optionally month and day)
setHours()
Set the hour (0-23)
setMilliseconds()
Set milliseconds (0-999)
setMinutes()
Sets the minutes (0-59)
setMonth()
Set the month (0-11)
setSeconds()
Sets the seconds (0-59)
setTime()
Set the time (milliseconds since January 1, 1970)
setUTCDate()
Sets the day of the month for a specified date according to universal time (also available for day, month, full year, hours, minutes etc.)
DOM Node
This allows JavaScript to update the web page and include node properties, node methods, and element methods.
attributes
Returns a live collection of all attributes registered to and element
baseURI
Provides the absolute base URL of an HTML element
childNodes
Gives a collection of an element’s child nodes
firstChild
Returns the first child node of an element
lastChild
The last child node of an element
nextSibling
Gives you the next node at the same node tree level
nodeName
Returns the name of a node
nodeType
Returns the type of a node
nodeValue
Sets or returns the value of a node
ownerDocument
The top-level document object for this node
parentNode
Returns the parent node of an element
previousSibling
Returns the node immediately preceding the current one
textContent
Sets or returns the textual content of a node and its descendants
appendChild()
Adds a new child node to an element as the last child node
cloneNode()
Clones a HTML element
compareDocumentPosition()
Compares the document position of two elements
getFeature()
Returns an object which implements the APIs of a specified feature
hasAttributes()
Returns true if an element has any attributes, otherwise false
hasChildNodes()
Returns true if an element has any child nodes, otherwise false
insertBefore()
Inserts a new child node before a specified, existing child node
isDefaultNamespace()
Returns true if a specified namespaceURI is the default, otherwise false
isEqualNode()
Checks if two elements are equal
isSameNode()
Checks if two elements are the same node
isSupported()
Returns true if a specified feature is supported on the element
lookupNamespaceURI()
Returns the namespaceURI associated with a given node
lookupPrefix()
Returns a DOMString containing the prefix for a given namespaceURI, if present
normalize()
Joins adjacent text nodes and removes empty text nodes in an element
removeChild()
Removes a child node from an element
replaceChild()
Replaces a child node in an element
getAttribute()
Returns the specified attribute value of an element node
getAttributeNS()
Returns string value of the attribute with the specified namespace and name
getAttributeNode()
Gets the specified attribute node
getAttributeNodeNS()
Returns the attribute node for the attribute with the given namespace and name
getElementsByTagName()
Provides a collection of all child elements with the specified tag name
getElementsByTagNameNS()
Returns a live HTMLCollection of elements with a certain tag name belonging to the given namespace
hasAttribute()
Returns true if an element has any attributes, otherwise false
hasAttributeNS()
Provides a true/false value indicating whether the current element in a given namespace has the specified attribute
removeAttribute()
Removes a specified attribute from an element
removeAttributeNS()
Removes the specified attribute from an element within a certain namespace
removeAttributeNode()
Takes away a specified attribute node and returns the removed node
setAttribute()
Sets or changes the specified attribute to a specified value
setAttributeNS()
Adds a new attribute or changes the value of an attribute with the given namespace and name
setAttributeNode()
Sets or changes the specified attribute node
setAttributeNodeNS()
Adds a new namespaced attribute node to an element
Events
These are the updates caused by JavaScript code and include mouse, keyboard, frame, form, drag, clipboard, media, animation, and other.
onclick
The event occurs when the user clicks on an element
oncontextmenu
User right-clicks on an element to open a context menu
ondblclick
The user double-clicks on an element
onmousedown
The user presses a mouse button over an element
onmouseenter
The pointer moves onto an element
onmouseleave
The pointer moves out of an element
onmousemove
The pointer is moving while it is over an element
onmouseover
When the pointer is moved onto an element or one of its children
onmouseout
The user moves the mouse pointer out of an element or one of its children
onmouseup
The user releases a mouse button while over an element
onkeydown
When the user is pressing a key down
onkeypress
The moment the user starts pressing a key
onkeyup
The user releases a key
onabort
The loading of a media is aborted
onbeforeunload
Event occurs before the document is about to be unloaded
onerror
An error occurs while loading an external file
onhashchange
There have been changes to the anchor part of a URL
onload
When an object has loaded
onpagehide
The user navigates away from a webpage
onpageshow
When the user navigates to a webpage
onresize
The document view is resized
onscroll
An element’s scrollbar is being scrolled
onunload
Event occurs when a page has unloaded
onblur
When an element loses focus
onchange
The content of a form element changes (for <input>, <select>and <textarea>)
onfocus
An element gets focus
onfocusin
When an element is about to get focus
onfocusout
The element is about to lose focus
oninput
User input on an element
oninvalid
An element is invalid
onreset
A form is reset
onsearch
The user writes something in a search field (for <input="search">)
onselect
The user selects some text (for <input> and <textarea>)
onsubmit
A form is submitted
ondrag
An element is dragged
ondragend
The user has finished dragging the element
ondragenter
The dragged element enters a drop target
ondragleave
A dragged element leaves the drop target
ondragover
The dragged element is on top of the drop target
ondragstart
User starts to drag an element
ondrop
Dragged element is dropped on the drop target
oncopy
User copies the content of an element
oncut
The user cuts an element’s content
onpaste
A user pastes content in an element
onabort
Media loading is aborted
oncanplay
The browser can start playing media (e.g. a file has buffered enough)
oncanplaythrough
When browser can play through media without stopping
ondurationchange
The duration of the media changes
onended
The media has reached its end
onerror
Happens when an error occurs while loading an external file
onloadeddata
Media data is loaded
onloadedmetadata
Meta Metadata (like dimensions and duration) are loaded
onloadstart
The browser starts looking for specified media
onpause
Media is paused either by the user or automatically
onplay
The media has been started or is no longer paused
onplaying
Media is playing after having been paused or stopped for buffering
onprogress
Browser is in the process of downloading the media
onratechange
The playing speed of the media changes
onseeked
The user is finished moving/skipping to a new position in the media
onseeking
The user starts moving/skipping
onstalled
The browser is trying to load the media but it is not available
onsuspend
Browser is intentionally not loading media
ontimeupdate
The playing position has changed (e.g. because of fast forward)
onvolumechange
Media volume has changed (including mute)
onwaiting
Media paused but expected to resume (for example, buffering)
animationend
A CSS animation is complete
animationiteration
CSS animation is repeated
animationstart
CSS animation has started
transitionend
Fired when a CSS transition has been completed
Errors
onmessage
A message is received through the event source
onoffline
The browser starts to work offline
ononline
The browser starts to work online
onpopstate
When the window’s history changes
onshow
An <menu> element is shown as a context menu
onstorage
A Web Storage area is updated
ontoggle
The user opens or closes the <details> element
onwheel
The mouse wheel rolls up or down over an element
ontouchcancel
Screen touch is interrupted
ontouchend
User finger is removed from a touch screen
ontouchmove
A finger is dragged across the screen
ontouchstart
Finger is placed on touch screen
try
Lets you define a block of code to test for errors
catch
Set up a block of code to execute in case of an error
throw
Create custom error messages instead of the standard JavaScript errors
finally
Lets you execute code, after try and catch, regardless of the result
name
Sets or returns the error name
message
Sets or returns an error message in string from
EvalError
An error has occurred in the eval() function
RangeError
A number is “out of range”
ReferenceError
An illegal reference has occurred
SyntaxError
A syntax error has occurred
TypeError
A type error has occurred
URIError
An encodeURI() error has occurred
Window Properties
closed
Checks whether a window has been closed or not and returns true or false
defaultStatus
Sets or returns the default text in the status bar of a window
document
Returns the document object for the window
frames
Returns all <iframe> elements in the current window
history
Provides the History object for the window
innerHeight
The inner height of a window’s content area
innerWidth
The inner width of the content area
length
Find out the number of <iframe> elements in the window
location
Returns the location object for the window
name
Sets or returns the name of a window
navigator
Returns the Navigator object for the window
opener
Returns a reference to the window that created the window
outerHeight
The outer height of a window, including toolbars/ scrollbars
outerWidth
The outer width of a window, including toolbars/ scrollbars
pageXOffset
Number of pixels the current document has been scrolled horizontally
pageYOffset
Number of pixels the document has been scrolled vertically
parent
The parent window of the current window
screen
Returns the Screen object for the window
screenLeft
The horizontal coordinate of the window (relative to the screen)
screenTop
The vertical coordinate of the window
screenX
Same as screenLeft but needed for some browsers
screenY
Same as screenTop but needed for some browsers
self
Returns the current window
status
Sets or returns the text in the status bar of a window
top
Returns the topmost browser window
alert()
Displays an alert box with a message and an OK button
blur()
Removes focus from the current window
clearInterval()
Clears a timer set with setInterval()
clearTimeout()
Clears a timer set with setTimeout()
close()
Closes the current window
confirm()
Displays a dialogue box with a message and an OK and Cancel button
focus()
Sets focus to the current window
moveBy()
Moves a window relative to its current position
moveTo()
Moves a window to a specified position
open()
Opens a new browser window
print()
Prints the content of the current window
prompt()
Displays a dialogue box that prompts the visitor for input
resizeBy()
Resizes the window by the specified number of pixels
resizeTo()
Resizes the window to a specified width and height
scrollBy()
Scrolls the document by a specified number of pixels
scrollTo()
Scrolls the document to specific coordinates
setInterval()
Calls a function or evaluates an expression at specified intervals
setTimeout()
Calls a function or evaluates an expression after a specified interval
stop()
Stops the window from loading
availHeight
Returns the height of the screen (excluding the Windows Taskbar)
availWidth
Returns the width of the screen (excluding the Windows Taskbar)
colorDepth
Returns the bit depth of the color palette for displaying images
height
The total height of the screen
pixelDepth
The color resolution of the screen in bits per pixel
width
The total width of the screen
Access this complete list in our downloadable reference chart.
How to Identify JavaScript Issues
JavaScript errors are common, and you should address them as soon as you can. Even if there are no coding errors, there may be issues with your JavaScript that cause slow page load times, such as unminifed JavaScript.
You can identify these issues by performing an audit of your site. Third-party tools like the Site Audit tool can identify issues like unminified JavaScript and site speed so that you can work on fixing them.
To use the Site Audit tool, you’ll need to create a project for your domain. If you already have a project created for your domain, read further to learn how to configure and run the tool.
Start by logging into your Semrush account. If you don’t have an existing account, you can create a free account. Just remember that the Site Audit provides limited functionality for Free Accounts.
Once you’re in, select “Dashboard” under “Management” to be taken to your project dashboard:
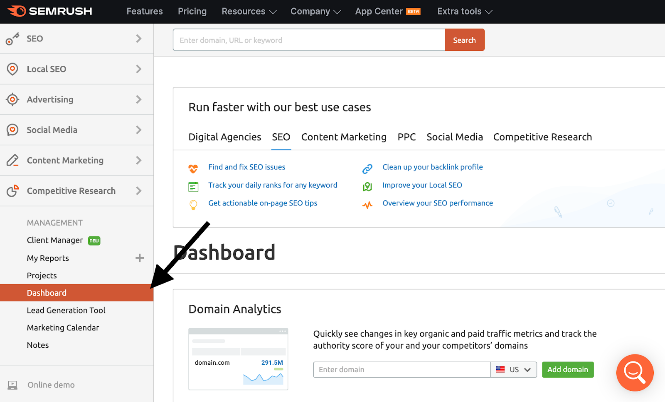
If you already have a project set up for your domain, you’ll see your project dashboard. Select the “Site Audit” card at the top of the page:
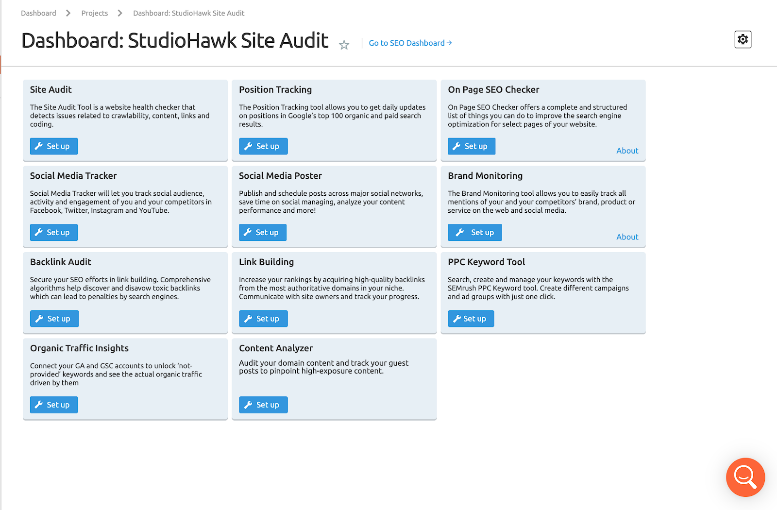
Once the tool is open, you’ll need to configure the audit’s settings, including the crawl scope, any website restrictions, and more. Once you’re happy with the settings, select “Start Site Audit:”
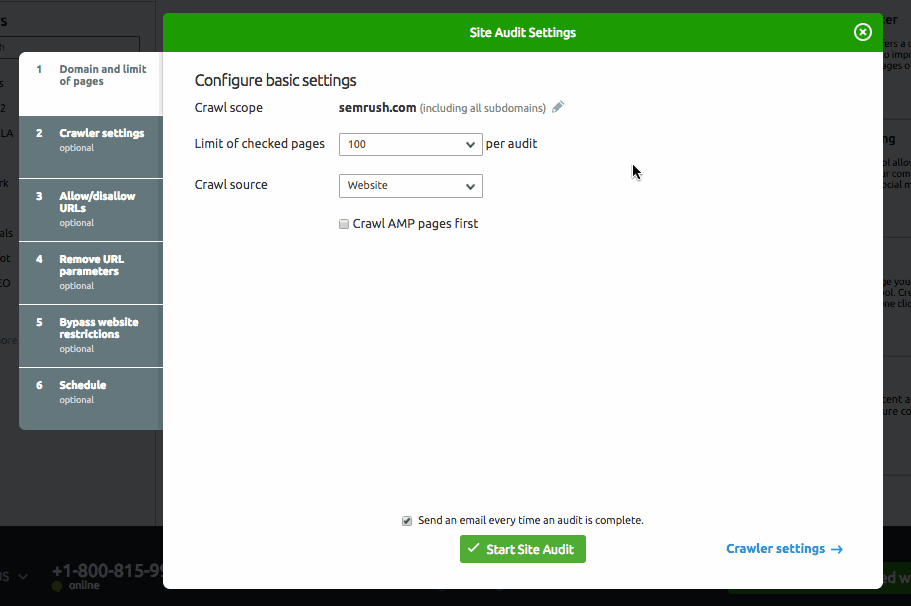
Once the audit is completed, you can navigate to the Site Audit’s dashboard for a complete overview of your audit. Navigate to the Issues tab for a list of site issues.
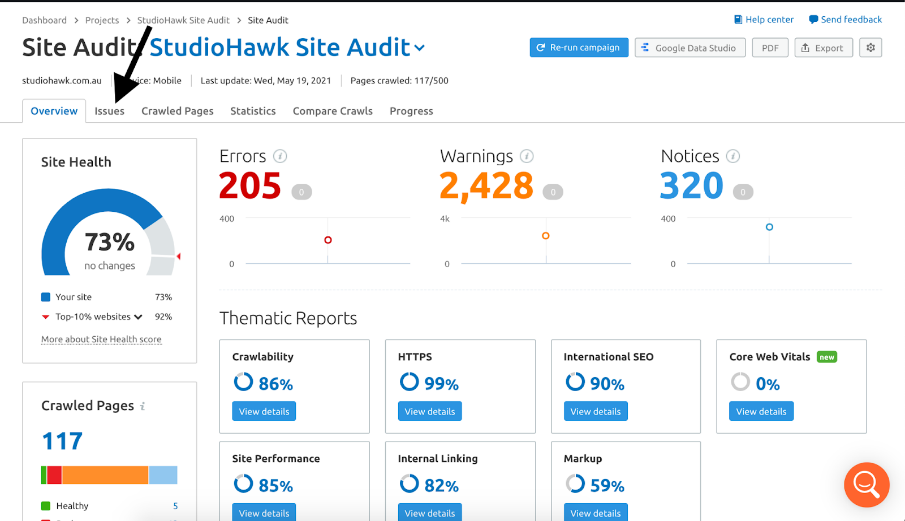
From there, you can filter the issues for any related to unminified JavaScript:
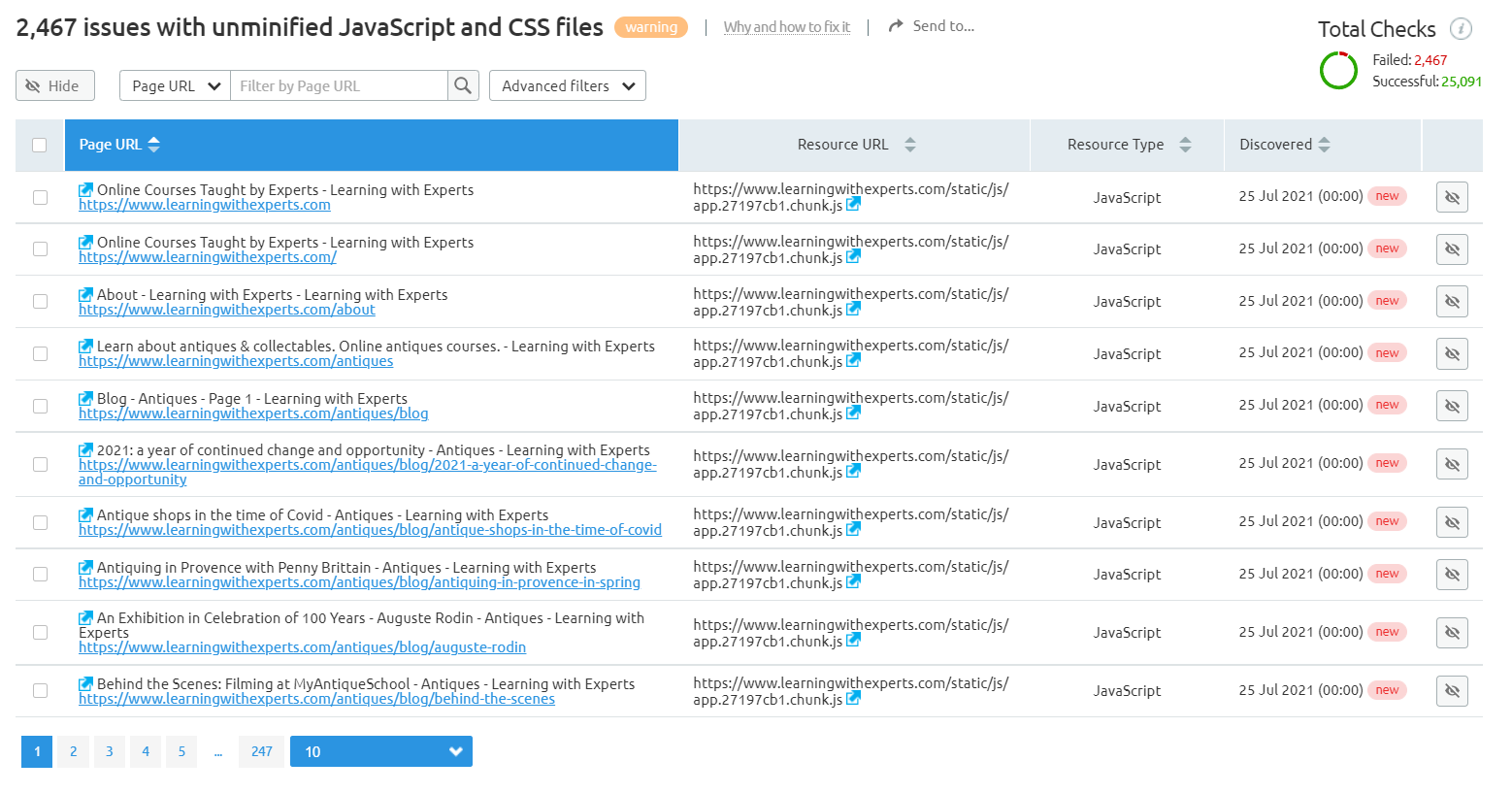
Although search engines are not able to render and see JavaScript elements, fixing JS-related issues like page speed can help you perform better in search engine rankings.
Final Thoughts
JavaScript is an important coding language that can help you transform the feel and function of your web pages. More importantly, good JavaScript code can help your website’s rankings by maintaining or improving page load speed.
Use our cheat sheet above to keep track of the most common JavaScript commands, and try third-party tools like the Site Audit to stay on top of your Javascript code when necessary.
Innovative SEO services
SEO is a patience game; no secret there. We`ll work with you to develop a Search strategy focused on producing increased traffic rankings in as early as 3-months.
A proven Allinclusive. SEO services for measuring, executing, and optimizing for Search Engine success. We say what we do and do what we say.
Our company as Semrush Agency Partner has designed a search engine optimization service that is both ethical and result-driven. We use the latest tools, strategies, and trends to help you move up in the search engines for the right keywords to get noticed by the right audience.
Today, you can schedule a Discovery call with us about your company needs.
Source:
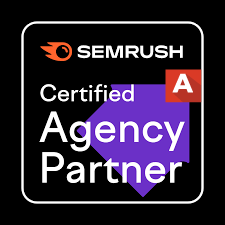